
Flutter 创建自定义路由过渡动画
TL;DR
- 使用
PageRouteBuilder
创建自定义路由 - 在
transitionsBuilder
方法里创建过渡动画 - 过渡动画示例
- 定义全局路由过渡动画
- 封装自定义路由
前言
在 Flutter
中使用 Navigator.of(context).push(Route route);
方法进行路由跳转时就需要传一个 Route
对象,通常使用 MaterialPageRoute(builder: () {});
创建,使用时会在路由跳转过程中添加默认的过渡动画。当需要自定义路由过渡动画时,就要使用 PageRouteBuilder
,它是 Flutter
提供的用来创建自定义的路由的一个类,实例化这个类会得到一个路由对象 Route
,要做的就是创建一个自定义的 Route
。
PageRouteBuilder
使用 PageRouteBuilder
创建自定义路由过渡动画时需要传入两个回调函数作为参数,一个必要参数 pageBuilder
,这个函数用来创建跳转的页面,另一个函数 transitionsBuilder
,这个函数就是实现过渡动画的地方。
transitionsBuilder
的child
参数是pageBuilder
函数返回的一个transitionsBuilder widget
部件,pageBuilder
方法仅会在第一次构建路由的时候被调用,Flutter
能够自动避免做额外的工作,整个过渡期间child
保存了同一个实例。
1 | PageRouteBuilder( |
创建自定义路由需要继承 PageRouteBuilder
,然后实现自定义路由的构造函数。
1 | // 定义 |
示例
使用 FirstPage
和 SecondPage
两个页面展示效果
1 | import 'package:flutter/material.dart'; |
FadeTransition
1 | class FadeRoute extends PageRouteBuilder { |
ScaleTransition
1 | class ScaleRoute extends PageRouteBuilder { |
RotationTransition
1 | class RotationRoute extends PageRouteBuilder { |
ScaleRotationRoute
结合两个过渡动画
1 | class ScaleRotationRoute extends PageRouteBuilder { |
TransformRoute
使用 Transform
部件创造 3D
效果
1 | import 'dart:math' show pi; |
EnterExitRoute
同时为进入页面和退出页面添加动画
1 | class EnterExitRoute extends PageRouteBuilder { |
使用 Navigator.pushNamed
方法跳转
在 onGenerateRoute
对跳转路由的 name
进行判断,对特定的路由添加过渡动画。
1 | class MyApp extends StatelessWidget { |
设置全局的路由过渡动画
Flutter
的默认路由过渡动画是由 buildTransitions
方法创建的,它使用的是 Theme.of(context).pageTransitionsTheme
方法,因此可以定义全局的路由跳转过渡动画。
1 |
|
首先自定义一个 TransitionBuilder
, buildTransitions
方法返回跳转页面。然后配置 theme
的 pageTransitionsTheme
,设置对应的平台,最后在使用 MaterialPageRoute
或者 CupertinoPageRoute
进行页面跳转时就会有自定义的过渡动画了。
1 | class ScaleTransitionBuilder extends PageTransitionsBuilder { |
将动画封装成一个库
将自定义的路由过渡动画封装起来方便使用。
1 | enum TransitionType { |
参考文章
Everything you need to know about Flutter page route transition
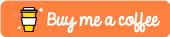